Skip to main content
Mastering Python
Take your skills to the next level with courses on the most popular programming languages Python. As the adoption of open source continues to rise, the demand for Python coding skills will also continue to grow. Learn one of today’s most popular programming languages from our technical experts, whether you’re new to using Python or want to learn more specific advanced functionalities of Python.
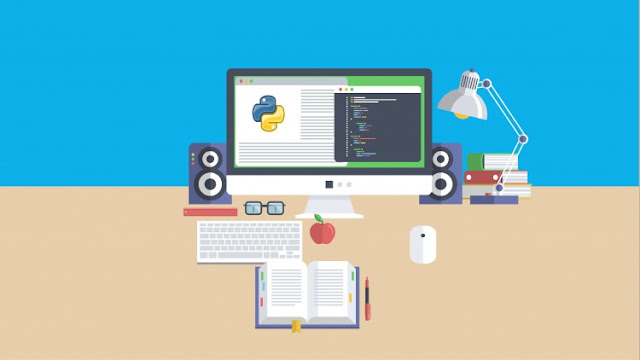
CHAPTER 1:INTRODUCTION
- An Introduction to Python
CHAPTER 2:BEGINNING PYTHON BASICS
- The print statement
- Comments
- Python Data Structures & Data Types
- String Operations in Python
- Simple Input & Output
- Simple Output Formatting
CHAPTER 3:PYTHON PROGRAM FLOW
- Indentation
- The If statement and its’ related statement
- An example with if and it’s related statement
- The while loop
- The for loop
- The range statement
- Break & Continue
- Assert
- Examples for looping
CHAPTER 4:FUNCTIONS & MODULES
- Create your own functions
- Functions Parameters
- Variable Arguments
- Scope of aFunction
- FunctionDocumentation/Docstrings
- Lambda Functions & map
- An Exercise with functions
- Create a Module
- Standard Modules
CHAPTER 5:EXCEPTIONS
- Errors
- Exception Handling with try
- Handling Multiple Exceptions
- Writing your own Exceptions
CHAPTER 6:FILE HANDLING
- File Handling Modes
- Reading Files
- Writing & Appending to Files
- Handling File Exceptions
- The with statement
CHAPTER 7:CLASSES IN PYTHON
- New Style Classes
- Creating Classes
- Instance Methods
- Inheritance
- Polymorphism
- Exception Classes & Custom Exceptions
CHAPTER 8:REGULAR EXPRESSIONS
- Simple Character Matches
- Special Characters
- Character Classes
- Quantifiers
- The Dot Character
- Greedy Matches
- Grouping
- Matching at Beginning or End
- Match Objects
- Substituting
- Splitting a String
- Compiling Regular Expressions
- Flags
CHAPTER 9:DATA STRUCTURES
- List Comprehensions
- Nested List Comprehensions
- Dictionary Comprehensions
- Functions
- Default Parameters
- Variable Arguments
- Specialized Sorts
- Iterators
- Generators
- The Functions any and all
- The with Statement
- Data Compression
CHAPTER 10:WRITING GUIS IN PYTHON
- Introduction
- Components and Events
- An Example GUI
- The root Component
- Adding a Button
- Entry Widgets
- Text Widgets
- Checkbuttons
- Radiobuttons
- Listboxes
- Frames
- Menus
- Binding Events to Widgets
CHAPTER 11:NETWORK PROGRAMMING
- Introduction
- A Daytime Server
- Clients and Servers
- The Client Program
- The Server Program
- Recap
- An Evaluation Client and Server
- The Server Portion
- A Threaded Server
CHAPTER 12:PYTHON MYSQL DATABASE ACCESS
- Introduction
- Installation
- DB Connection
- Creating DB Table
- INSERT, READ,UPDATE, DELETE operations
- COMMIT & ROLLBACK operation
- Handling Errors
Comments
Post a Comment